Engineering
React is a popular front-end library for building web applications. It has become the go-to choice for many web developers due to its reusability, scalability, and maintainability. However, as the project grows, it becomes challenging to manage the codebase. In this article, we will discuss some of the best practices for front-end code organization and architecture in a React application.
Folder structure
Folder structure is one of the most critical aspects of code organization. A well-organized folder structure makes it easier to find and maintain your code. A good folder structure contributes to the overall maintainability of the codebase, making it easier for team members to work on the project.
The structure should be intuitive and easy to navigate. In a React application, the typical folder structure consists of three main folders:
- Components: This folder contains all the reusable components in your application. It is a good practice to organize components in their respective folders with all the necessary files. This helps to keep the components organized and easy to find.
- Pages: This folder contains all the pages of your application. Each page should have its folder with the corresponding JavaScript and CSS files. This helps to keep the pages organized and easy to maintain.
- Utils: This folder contains all the utility functions, such as API calls or authentication logic. This helps to keep the functions organized and easy to find.
As the project scales further, it often makes sense to make Feature folders for each feature that lives in your application.
For example:
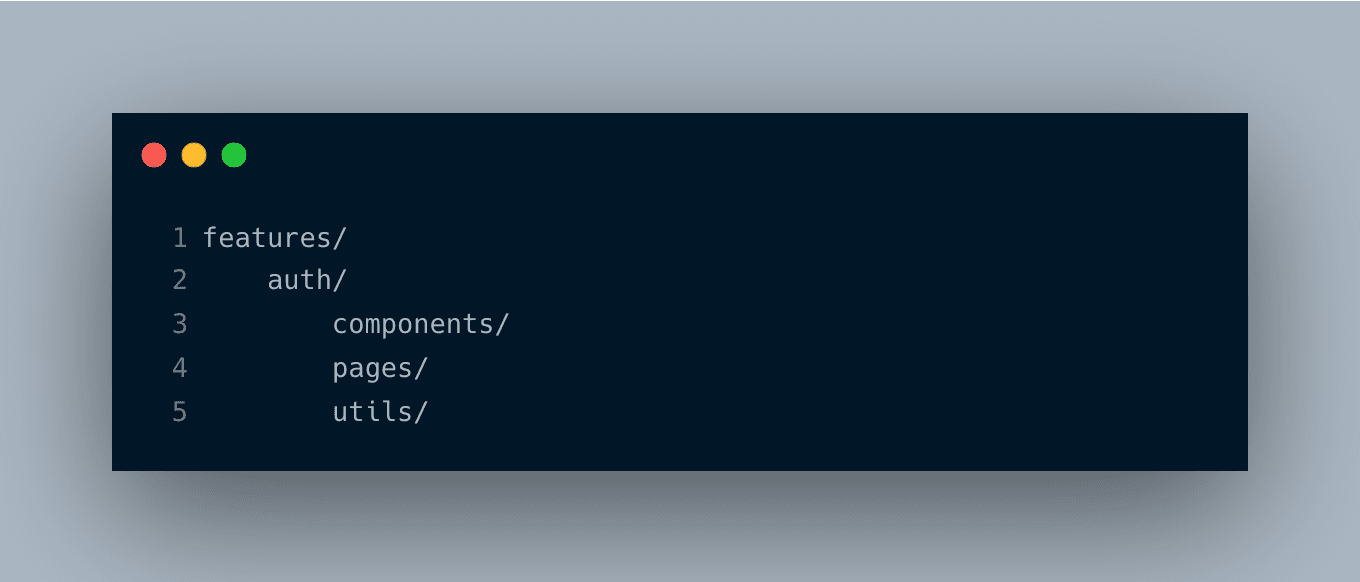
Naming conventions
Good naming conventions make your code more readable and understandable. Naming conventions are one of the most critical aspects of code organization, and they contribute to the overall maintainability of the codebase.
In a React application, naming conventions play a crucial role in code organization, readability, and maintainability. By following these best practices, your code can be more understandable and easier to maintain.
Firstly, components and folders should be named using PascalCase. It means that the first letter of each word should be capitalized. This naming convention helps to distinguish the components from the other elements in your codebase. Furthermore, it makes it easier to differentiate between components and other elements that are named using camelCase.
Secondly, files should be named using camelCase. It means that the first letter should be lowercase, and the first letter of every subsequent word should be capitalized. This naming convention helps to distinguish between different files and makes it easier to find the right file when you need it.
Thirdly, when naming components, it is important to choose descriptive and meaningful names. This practice helps to make your code more readable and understandable. For example, instead of naming a component Card, you can name it UserCard or ProductCard. It helps to indicate the purpose of the component and makes it easier to understand its usage.
Lastly, avoid using generic names like Box or Wrapper. These names are not descriptive and can cause confusion when working with your codebase. It is better to use more specific names that indicate the purpose of the component.
By following these naming conventions, you can make your code more readable, understandable, and maintainable. It is an essential part of front-end code organization and architecture in a React application.
Component architecture
A solid component architecture is essential for software maintainability and reuse. To achieve this, it's important to separate concerns and assign specific tasks to each component. This simplifies code maintenance and makes it easier to reuse the code across multiple projects.
When building a React application, there are several best practices that can help create a good component architecture. One such practice is to keep components small and focused on a single responsibility. This means that each component should perform one task effectively. For example, using presentational components for rendering UI elements and container components for managing state and passing props to other components is also a good practice.
Another good practice is to use higher-order components (HOCs) for cross-cutting concerns like authentication or data fetching. HOCs can help reduce code duplication and improve maintainability. Similarly, using render props or hooks to share code between components can also be beneficial.
By following these best practices, you can create a scalable, maintainable, and reusable codebase that can be easily adapted and extended as needed. This approach not only reduces development time and costs, but also ensures that the software is of the highest quality and meets the needs of users.
Code style
Maintaining a consistent code style can greatly improve the readability and understandability of your code. In a React application, it's recommended to adhere to the following best practices:
- Implement a linter, such as ESLint, to ensure a uniform code style.
- Favor functional components over class components.
- Utilize destructuring to extract values from props and state.
- Use arrow functions for event handlers.
- Apply template literals for string interpolation.
By adopting these best practices, you can enhance the clarity, comprehensibility, and maintainability of your code.
State management
State management is a crucial part of a React application. It is essential to keep the state organized and up-to-date. When we talk about state in React, we mean the data that determines the behavior of the UI. As your application grows, managing the state can become increasingly difficult.
A good state management system can make your application more scalable and maintainable. It can help you manage complex state logic, handle interactions between components, and keep the codebase organized.
There are several state management libraries available for React, including Redux, MobX, and Context API. Each library has its own strengths and weaknesses, and it is best to choose a state management system that suits your project requirements.
Redux is a popular option that provides a centralized store for all application state. It follows a unidirectional data flow and provides a set of tools for handling complex state logic. MobX is another popular library that uses observables to manage state. It provides a simpler API than Redux and is easier to learn. Context API is a built-in React feature that provides a way to share state between components without the need for a third-party library. It is useful for small to medium-sized applications that do not require complex state management.
Separating client state and server state is essential for building scalable React applications. Client state refers to the data that is stored on the client-side, such as user preferences, form data, or UI state. Server state, on the other hand, refers to the data that is stored on the server-side, such as user authentication, database records, or API responses. Common libraries that are good for managing server state are useSWR and React Query.
In summary, state management is a crucial aspect of building scalable and maintainable React applications. By choosing the right state management system for your project and using it effectively, you can ensure that your application's state is organized, up-to-date, and easy to manage.
Wrapping up
In conclusion, front-end code organization and architecture in a React application is crucial for building scalable, maintainable, and reusable codebases. By following best practices such as having a well-organized folder structure, good naming conventions, a good component architecture, consistent code style, a good state management system, and proper testing and deployment, you can ensure that your application is easy to maintain and scalable as it grows. It may take some time to implement these best practices, but they will pay off in the long run, making it easier for team members to work on the project and build robust and scalable React applications.